Project configuration¶
Normally, the installer limits the modifications to be performed in the configuration files and your application is operational after installation
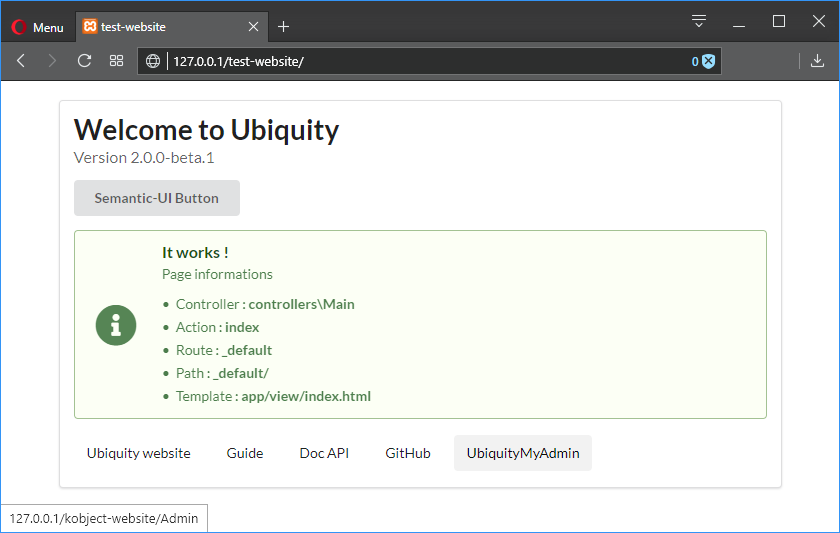
Main configuration¶
The main configuration of a project is localised in the app/conf/config.php
file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | return array(
"siteUrl"=>"%siteUrl%",
"database"=>[
"dbName"=>"%dbName%",
"serverName"=>"%serverName%",
"port"=>"%port%",
"user"=>"%user%",
"password"=>"%password%"
],
"namespaces"=>[],
"templateEngine"=>'Ubiquity\views\engine\twig\Twig',
"templateEngineOptions"=>array("cache"=>false),
"test"=>false,
"debug"=>false,
"di"=>[%injections%],
"cacheDirectory"=>"cache/",
"mvcNS"=>["models"=>"models","controllers"=>"controllers"]
);
|
Services configuration¶
Services loaded on startup are configured in the app/conf/services.php
file.
1 2 3 4 5 6 7 8 9 10 | use Ubiquity\controllers\Router;
try{
\Ubiquity\cache\CacheManager::startProd($config);
}catch(Exception $e){
//Do something
}
\Ubiquity\orm\DAO::startDatabase($config);
Router::start();
Router::addRoute("_default", "controllers\\IndexController");
|
Pretty URLs¶
Apache¶
The framework ships with an .htaccess file that is used to allow URLs without index.php. If you use Apache to serve your Ubiquity application, be sure to enable the mod_rewrite module.
AddDefaultCharset UTF-8
<IfModule mod_rewrite.c>
RewriteEngine On
RewriteBase /blog/
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{HTTP_ACCEPT} !(.*images.*)
RewriteRule ^(.*)$ index.php?c=$1 [L,QSA]
</IfModule>
See Apache configuration for more.
Nginx¶
On Nginx, the following directive in your site configuration will allow “pretty” URLs:
location /{
rewrite ^/(.*)$ /index.php?c=$1 last;
}
See NginX configuration for more.
Laravel Valet Driver¶
Valet is a php development environment for Mac minimalists. No Vagrant
, no /etc/hosts
file. You can even share your sites publicly using local tunnels.
Laravel Valet configures your Mac to always run Nginx
in the background when your machine starts. Then, using DnsMasq
, Valet proxies all requests on the *.test
domain to point to sites installed on your local machine.
Get more info about Laravel Valet
Create UbiquityValetDriver.php
under ~/.config/valet/Drivers/
add below php code and save it.
<?php
class UbiquityValetDriver extends BasicValetDriver{
/**
* Determine if the driver serves the request.
*
* @param string $sitePath
* @param string $siteName
* @param string $uri
* @return bool
*/
public function serves($sitePath, $siteName, $uri){
if(is_dir($sitePath . DIRECTORY_SEPARATOR . '.ubiquity')) {
return true;
}
return false;
}
public function isStaticFile($sitePath, $siteName, $uri){
if(is_file($sitePath . $uri)) {
return $sitePath . $uri;
}
return false;
}
/**
* Get the fully resolved path to the application's front controller.
*
* @param string $sitePath
* @param string $siteName
* @param string $uri
* @return string
*/
public function frontControllerPath($sitePath, $siteName, $uri){
$sitePath.='/public';
$_SERVER['DOCUMENT_ROOT'] = $sitePath;
$_SERVER['SCRIPT_NAME'] = '/index.php';
$_SERVER['SCRIPT_FILENAME'] = $sitePath . '/index.php';
$_SERVER['DOCUMENT_URI'] = $sitePath . '/index.php';
$_SERVER['PHP_SELF'] = '/index.php';
$_GET['c'] = '';
if($uri) {
$_GET['c'] = ltrim($uri, '/');
$_SERVER['PHP_SELF'] = $_SERVER['PHP_SELF']. $uri;
$_SERVER['PATH_INFO'] = $uri;
}
$indexPath = $sitePath . '/index.php';
if(file_exists($indexPath)) {
return $indexPath;
}
}
}